【Minecraft】見張り台を作る
2020年5月16日
お城には敵の来襲を監視する見張り台が不可欠です。これも追加してみましょう。まずは、床を作って、その周りを城壁と同じようにぐるっと壁を作ります。
こんな感じのコードになります。
import mcpi.minecraft as minecraft
import mcpi.block as block
import server
import sys
mc = minecraft.Minecraft()
mc.postToChat("Wall!")
playerPos = mc.player.getPos()
mc.player.setRotation(0)
width = 41
height = 6
org_x = playerPos.x + 5
org_y = playerPos.y
org_z = playerPos.z + 5
# X軸側の壁(手前)
for y in range(0, height):
for x in range(1, width):
mc.setBlock(
org_x + x,
org_y + y,
org_z,
block.STONE_BRICK)
for x in range(1, width, 2):
mc.setBlock(
org_x + x,
org_y + height,
org_z,
block.STONE_BRICK)
for x in range(2, width, 2):
mc.setBlock(
org_x + x,
org_y + height,
org_z,
block.TORCH)
# X軸側の壁(奥)
for y in range(0, height):
for x in range(1, width):
mc.setBlock(
org_x + x,
org_y + y,
org_z + width,
block.STONE_BRICK)
for x in range(1, width, 2):
mc.setBlock(
org_x + x,
org_y + height,
org_z + width,
block.STONE_BRICK)
for x in range(2, width, 2):
mc.setBlock(
org_x + x,
org_y + height,
org_z + width,
block.TORCH)
# Z軸側の壁(手前)
for y in range(0, height):
for z in range(1, width):
mc.setBlock(
org_x,
org_y + y,
org_z + z,
block.STONE_BRICK)
for z in range(1, width, 2):
mc.setBlock(
org_x,
org_y + height,
org_z + z,
block.STONE_BRICK)
for z in range(2, width, 2):
mc.setBlock(
org_x,
org_y + height,
org_z + z,
block.TORCH)
# Z軸側の壁(奥)
for y in range(0, height):
for z in range(1, width):
mc.setBlock(
org_x + width,
org_y + y,
org_z + z,
block.STONE_BRICK)
for z in range(1, width, 2):
mc.setBlock(
org_x + width,
org_y + height,
org_z + z,
block.STONE_BRICK)
for z in range(2, width, 2):
mc.setBlock(
org_x + width,
org_y + height,
org_z + z,
block.TORCH)
# 扉を作る
for y in range(0, height-2):
mc.setBlock(
org_x,
org_y + y,
org_z + int(width/2) - 1,
block.IRON_BLOCK)
mc.setBlock(
org_x,
org_y + y,
org_z + int(width/2),
block.IRON_BLOCK)
mc.setBlock(
org_x,
org_y + y,
org_z + int(width/2) + 1,
block.IRON_BLOCK)
# 塔を建てる
TOWER_width = 7
TOWER_height = 16
TOWER_OFFEST_x = int((width-TOWER_width)/2)
TOWER_OFFEST_z = int((width-TOWER_width)/2)
# X軸側の塔(手前)
for y in range(0, TOWER_height):
for x in range(1, TOWER_width):
mc.setBlock(
org_x + x + TOWER_OFFEST_x,
org_y + y,
org_z + TOWER_OFFEST_z,
block.STONE_BRICK)
# X軸側の塔(奥)
for y in range(0, TOWER_height):
for x in range(1, TOWER_width):
mc.setBlock(
org_x + x + TOWER_OFFEST_x,
org_y + y,
org_z + TOWER_width + TOWER_OFFEST_z,
block.STONE_BRICK)
# Z軸側の塔(手前)
for y in range(0, TOWER_height):
for z in range(1, TOWER_width):
mc.setBlock(
org_x + TOWER_OFFEST_x,
org_y + y,
org_z + z + TOWER_OFFEST_z,
block.STONE_BRICK)
# Z軸側の塔(奥)
for y in range(0, TOWER_height):
for z in range(1, TOWER_width):
mc.setBlock(
org_x + TOWER_width + TOWER_OFFEST_x,
org_y + y,
org_z + z + TOWER_OFFEST_x,
block.STONE_BRICK)
# 見張り台を作る
TERRACE_width = 10
TERRACE_height = 2
TERRACE_floor_height = 16
TERRACE_OFFEST_x = int((width-TERRACE_width)/2)
TERRACE_OFFEST_z = int((width-TERRACE_width)/2)
# 見張り台(床)
for x in range(1, TERRACE_width):
for z in range(1, TERRACE_width):
mc.setBlock(
org_x + x + TERRACE_OFFEST_x,
org_y + TERRACE_floor_height,
org_z + z + TERRACE_OFFEST_z,
block.STONE_BRICK)
# X軸側の見張り台(手前)
for y in range(0, TERRACE_height):
for x in range(1, TERRACE_width):
mc.setBlock(
org_x + x + TERRACE_OFFEST_x,
org_y + y + TERRACE_floor_height,
org_z + TERRACE_OFFEST_z,
block.STONE_BRICK)
# X軸側の見張り台(奥)
for y in range(0, TERRACE_height):
for x in range(1, TERRACE_width):
mc.setBlock(
org_x + x + TERRACE_OFFEST_x,
org_y + y + TERRACE_floor_height,
org_z + TERRACE_width + TERRACE_OFFEST_z,
block.STONE_BRICK)
# Z軸側の見張り台(手前)
for y in range(0, TERRACE_height):
for z in range(1, TERRACE_width):
mc.setBlock(
org_x + TERRACE_OFFEST_x,
org_y + y + TERRACE_floor_height,
org_z + z + TERRACE_OFFEST_z,
block.STONE_BRICK)
# Z軸側の見張り台(奥)
for y in range(0, TERRACE_height):
for z in range(1, TERRACE_width):
mc.setBlock(
org_x + TERRACE_width + TERRACE_OFFEST_x,
org_y + y + TERRACE_floor_height,
org_z + z + TERRACE_OFFEST_x,
block.STONE_BRICK)
mc.postToChat("Wall constructed!")
このコードをwall3.pyとして保存し、ゲーム画面のチャットから
/py wall3
と入力すると、次のような立派な見張り台ができます。
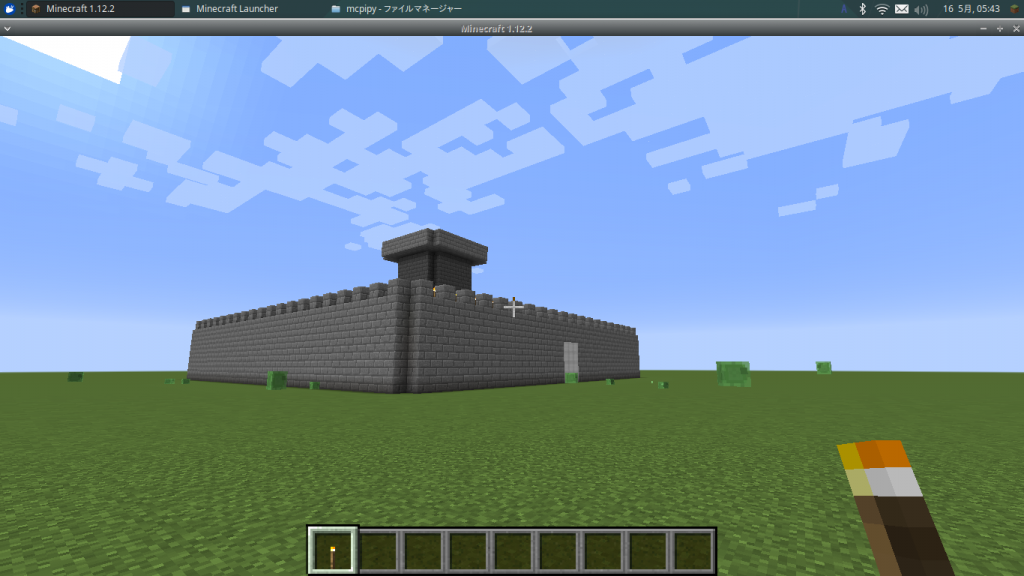